Author: Samuel Adeshina
Viewers: 498
Last month viewers: 3
Package: PHP Dependency Injection Container
Read this article to learn what is dependency injection and inversion of control and how it can be implemented in PHP to benefit your projects' structure.

Contents
Introduction
How Dependency Injection Works?
Dependency Injection in Practice
Dependency Injection and the Single Responsability Principle
Conclusion
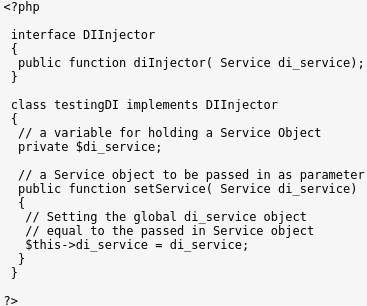
Introduction
Dependency Injection is an object oriented design pattern that you come across when extending application frameworks logic. It has to do with "more than one class (object) depending on the services of another".
There are lots of articles, tutorials, explanations on the Dependency Injection design pattern. They also mention Inversion of Control. It is based on the popularly known Hollywood catch phrase, "Don't call us, we'll call you".
Those articles often have two things in common: its either their base of explanation on a particular technology (such as Java or C#) or they delve into highly detailed concepts without explaining the main concepts behind the dependency design principle.
This article aims to bridge those gaps by explaining the main concepts behind Dependency Injection (Inversion of Control) design pattern, explain its advantages when compared with other alternatives and list the challenges that you may be faced when using it to write applications.
How Dependency Injection Works?
In normal everyday object oriented application development, a dependency object is passed to a dependent object directly. This may result in lots of issues such as tight coupling, less flexible and reusable code, among others.
Dependency Injection and Inversion of Control Containers are meant to solve these problems by making the dependent object (client) call a dependency when needed. This makes it very easy to develop robust, independent and reusable object oriented class services.
Dependency Injection in Practice
Let me illustrate these concepts with a quick example of a car class object that is dependent on an automobile class.
<?php
class automobile
{
public function __construct()
{
echo "I'm the Automobile Class";
}
public function doSomething()
{
echo "I'm doing something";
return;
}
}
?>
The snippet above defines the automobile class which would act as a parent to the car class. Normally, the car class object would be defined this way:
<?php class testingDI { // a variable for holding a Service Object private $di_service; public function __construct() { } // a Service object to be passed in as parameter public function setService( Service di_service) { // Setting the global di_service object // equal to the passed in Service object $this->di_service = di_service; } } ?>
Similarly, in interface injection, the client (dependent object) implements an interface which defines how a dependency should be accepted and set. An example is illustrated below:
<?php interface DIInjector { public function diInjector( Service di_service); } class testingDI implements DIInjector { // a variable for holding a Service Object private $di_service; // a Service object to be passed in as parameter public function setService( Service di_service) { // Setting the global di_service object // equal to the passed in Service object $this->di_service = di_service; } } ?>
Dependency Injection helps to create software that follows the dependency inversion principle. The Dependency Inversion Principle is a common way, especially with object oriented designs, of decoupling various part of a software module into individual entities.
Following this principle means that the Client (the dependent object) does not need to know how to construct it's dependencies, it's the injecting code that constructs the services (the dependencies) and injects them into the client
Dependency Injection and the Single Responsability Principle
This is very similar to the Single Responsibility Principle that states that every object (a class in this case) should have responsibility over a single part of the functionality provided by the software. In order words, the Single Responsibility Principle is all about the simple concept of One Class, One Task.
Conclusion
Dependency Injection is a software design pattern that helps to provide a better structure to an object oriented software design. Although, there are more advanced alternatives to pure Dependency Injection such as using a Dependency Injection (Inversion of Control) Container, instead of directly using the Dependency Injection approach, it still is a major building block for most of this concepts.
In the next article, we look further into the various ways of implementing Dependency Injection. We would talk more about the Controller Injection, Setter Injection and Interface Injection approaches and how they can be useful in various scenarios.
We will also talk about IoC Containers and how to build one for better software reusability, maintainability and testability.
If you liked this article or you have a question about the presented dependency injection concepts, post a comment here.
You need to be a registered user or login to post a comment
Login Immediately with your account on:
Comments:
4. No DI between car and automobile... - steven (2015-11-03 21:47)
No DI between car and automobile... but inheritance... - 2 replies
Read the whole comment and replies
3. Dependency Injection - Felix Ivan Romero Rodríguez (2015-11-03 19:09)
Ideas about... - 0 replies
Read the whole comment and replies
2. Car - Class analogy - Samuel Adeshina (2015-11-03 19:07)
its all about controlling how objects interacts with one another... - 0 replies
Read the whole comment and replies
1. Not clear because no car class definition - steven (2015-11-03 11:01)
Not clear because no car class definition... - 3 replies
Read the whole comment and replies